What Is an XML-RPC API and Why SaaS Teams Use Them
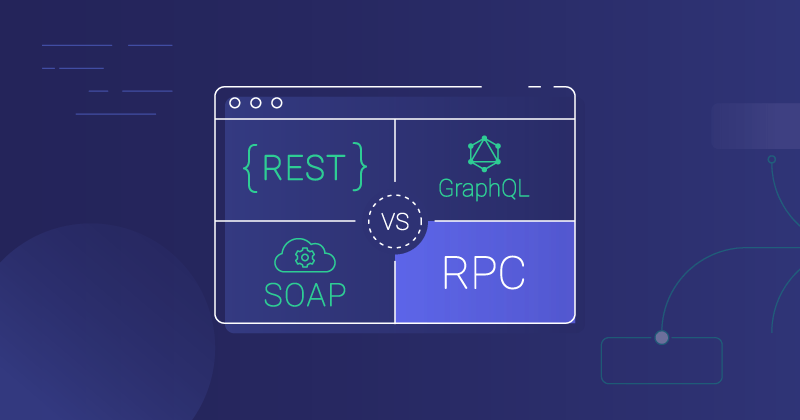
In a recent overview post, we addressed several API types (including RPC). In this post, we'll look at XML-RPC APIs in more detail.
An XML-RPC API is an API (application programming interface) that exchanges data via remote procedure calls. Let's break it down:
- Remote: A local procedure is in the same app or system as the code. A remote procedure is in a different app or system. For SaaS, the client (web app) calls a procedure on the server via the API.
- Procedure: A procedure is a subroutine or function in code. In many cases, it is code that can be run locally by an app or invoked remotely.
- Call: A call is a request from the client to the server.
XML RPC APIs use XML as the transport language (or data exchange format). XML stands for extensible markup language.
The history of RPC APIs
RPC APIs have been around in theory since the 1970s and in practice since the 1980s. And the idea of remotely invoking a function or subroutine has been around even longer.
Before REST APIs became popular for the web in about 2005, XML-RPC (born in 1998) was one of the more common web APIs. SOAP is an evolution of XML-RPC but has its own patterns.
Another web-based RPC is JSON-RPC (born in 2007). JSON-RPC uses JSON as its transport language. JSON-RPC APIs are used in scenarios where a server must rapidly process a high volume of messages. As a result, JSON-RPC is currently used for cryptocurrency blockchains.
While both XML-RPC and JSON-RPC commonly use HTTP as the transfer protocol for web implementations, only XML-RPC is restricted to that protocol. JSON-RPC can use various transfer protocols.
We should also mention gRPC APIs. Originally developed by Google, gRPC is now open source. While a relative newcomer to the field (initially released in 2015), gRPC has a growing number of companies using it, primarily to connect apps and services within closed systems such as phones and tablets.
We'll be focusing on XML-RPC APIs for the balance of this post since that is the RPC API type our customers are most likely to see in the course of building B2B SaaS integrations.
How XML-RPC APIs work and what they are used for
XML-RPC APIs use HTTP over the web to enable data import and export between apps. XML-RPC APIs only accept POST as a valid HTTP verb. XML-APIs have a single endpoint, unlike REST, which can have many endpoints.
XML-RPC APIs expose remote procedures to support standard CRUD (create, read, update, delete) operations and make it easy to execute non-CRUD functions.
XML-RPC APIs can use any of the standard approaches to authentication, including basic auth (not recommended), API keys (common), or OAuth 2.0 (best option).
Each message sent to an XML-RPC API is a request and results in a corresponding response. The response may be valid, or it may be a fault (error) response.
Though XML-RPC is based on XML, it uses a minimal XML vocabulary for requests and responses.
Each request includes the following tags:
- XML version (required)
- methodCall (required)
- methodName (required)
- params (optional)
- param (optional, required if params)
- value (optional, required if param)
Here's a sample XML-RPC request (without headers) where we request the account name for a specific customer ID:
<?xml version="1.0"?>
<methodCall>
<methodName>listAccounts</methodName>
<params>
<param>
<value><cuid>u6f6igwe3</cuid></value>
</param>
</params>
</methodCall>
Each response includes the following tags, all of which are required.
- XML version
- methodResponse
- params
- param
- value
Here's an example of an XML response (without headers):
<?xml version=1.0"?>
<methodResponse>
<params>
<param>
<value><string>Progix Rockets, Inc.</string></value>
</param>
</params>
</methodResponse>
Or, in the case of a fault, the following tags are used:
- XML version (required)
- methodResponse (required)
- fault (required)
- value (required)
- struct (required; includes the code and string)
Here's an example of an XML-RPC fault message (without headers):
<?xml version="1.0"?>
<methodResponse>
<fault>
<value>
<struct>
<member>
<name>faultCode</name>
<value><int>47</int></value>
</member>
<member>
<name>faultString</name>
<value><string>Method does not exist.</string></value>
</member>
</struct>
</value>
</fault>
</methodResponse>
Why SaaS teams and products use XML-RPC API
With REST APIs being designed specifically for the web and using many web conventions, why would teams use an XML-RPC API? One reason is that XML-RPC isn't limited to standard CRUD operations. As a result, if you have remote functions to calculate something, it will be much more straightforward to perform that function via an XML-RPC API than to do it via a REST API.
And because an XML-RPC API has a single endpoint, it can be more attractive to use XML-RPC instead of REST in a situation where tracking and managing many endpoints creates much extra work. For example, Odoo's SaaS app exposes 800 models (data collections) via an XML-RPC API. Each model has remote procedures that can be called via the API to perform CRUD and non-CRUD operations. If Odoo had implemented its API with REST, we would have more than 3,200 individual endpoints.
What are best practices for setting up RPC APIs?
XML-RPC APIs can be built in just about any programming language, though some common languages are PHP, Python, and Perl. Regardless of language, make sure your XML-RPC API is set up as follows:
- Only support the POST verb for requests. Using GET for XML-RPC is not recommended since you can't pass in a request body with GET.
Content-Type
should always betext/html
.- You should use a single response code (usually HTTP 200) for responses.
How do XML-RPC APIs work with integrations?
When you integrate with an XML-RPC API, you'll deal with the usual authentication tasks for any integration, such as handling API keys and creating OAuth 2.0 callback URLs. Once you have figured out auth, writing code to interact with the API is straightforward. Most modern languages have an XML-RPC library you can import (the NodeJS platform includes xmlrpc). Sample JavaScript code to connect with an XML-RPC API might look something like this:
// Import library
import xmlrpc from "xmlrpc";
// Create a generic client
const client = xmlrpc.createSecureClient({
host: "api.company.com",
port: 443,
path: "/xmlrpc-endpoint",
});
// Make a method call given a search parameter
client.methodCall(
"listAccounts",
[["accountId", "=", "abc-123"]],
function (error, value) {
// Results of the method response
console.log("Method response for 'listAccounts': " + value);
}
);
Your team can reuse the generic XML-RPC client pointed at the API's endpoint and can build requests with whatever methods and params you need.
For a refresher on APIs and integrations, check out API vs Integration: What is the Difference?
The future of XML-RPC APIs
While XML-RPC APIs are not used as commonly with web apps as certain other types (REST being the obvious example), they have their niche. For instance, XML-RPC APIs are well suited to executing non-CRUD remote procedures.
And using XML-RPC may be a good choice if going with another type of API (such as REST) would lead to an unwieldy number of endpoints.
For more content on XML-RPC APIs and other integration topics, check out our guide to API integrations.

Want to learn more about API integrations?
Download our API Integrations Guide to see what an API integration is and learn how it works.
Get my CopyAbout Prismatic
Prismatic, the world's most versatile embedded iPaaS, helps B2B SaaS teams launch powerful product integrations up to 8x faster. The industry-leading platform provides a comprehensive toolset so teams can build integrations fast, deploy and support them at scale, and embed them in their products so customers can self-serve. It encompasses both low-code and code-native building experiences, pre-built app connectors, deployment and support tooling, and an embedded integration marketplace. From startups to Fortune 100, B2B SaaS companies across a wide range of verticals and many countries rely on Prismatic to power their integrations.