What Is a SOAP API and Why SaaS Teams Use Them
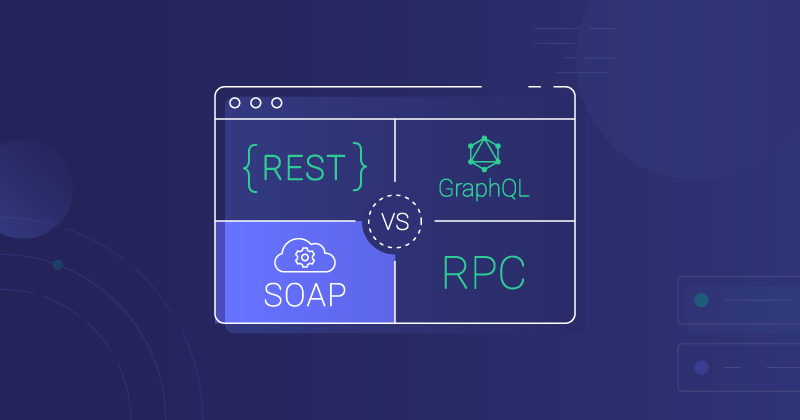
In a recent overview post, we addressed several API types (including SOAP). We have also examined XML-RPC APIs (which are a predecessor to SOAP APIs in many regards). In this post, we'll look at SOAP APIs in more detail.
A SOAP API (application programming interface) is an API that uses SOAP (simple object access protocol) to exchange data. As with an XML-RPC API, SOAP uses XML (extensible markup language) as its transport language or data exchange format and follows the RPC (remote procedure call) pattern common to RPC APIs.
SOAP APIs are, in fact, RPC APIs but are treated as a separate type of API because of the rules defined by the SOAP specification.
The history of SOAP APIs
Microsoft originally designed SOAP in 1998 to integrate web services agnostic of OS, framework, or language. It built on the earlier XML-RPC foundation, but took things much farther in terms of defining a standard.
SOAP can use several transport protocols, including HTTP (hypertext transport protocol), FTP (file transfer protocol), TCP (transmission control protocol), and SMTP (simple mail transfer protocol). Practically, however, HTTP/HTTPS is used for most SOAP API implementations. SOAP can use several transfer protocols, including HTTP (hypertext transfer protocol), FTP (file transfer protocol), TCP (transmission control protocol), and SMTP (simple mail transfer protocol). Practically, however, HTTP/HTTPS is used for most SOAP API implementations.
Though some SOAP APIs have been replaced with other APIs, such as REST, many SOAP APIs continue to support web integrations today.
How SOAP APIs work and what they are used for
SOAP APIs use HTTP (and other protocols, but mostly HTTP) over the web to enable data import and export between apps. Because SOAP APIs are technically RPC-APIs, they only accept POST as a valid HTTP method. And each SOAP API has a single endpoint, unlike a REST API, which can have many endpoints.
SOAP APIs expose remote procedures to support standard CRUD (create, read, update, delete) operations and non-CRUD operations.
SOAP also uses WSDL (web services description language), a subset of XML, to describe how to call the functions/procedures available via the API. You can read the WSDL file for a SOAP API to determine what you can do with the API, what kind of data you can pass to it, and what you can expect to get back from it. Expert tip – WSDL is pronounced as "wiz-dull."
SOAP APIs can use various types of authentication based on HTTP, including basic auth (not recommended), API keys (common), or OAuth 2.0 (best option). In addition, SOAP uses WS-Security for scenarios where HTTPS is deemed insufficient. WS-Security is an extension to SOAP that provides protection through message integrity, message confidentiality, and single message authentication.
Each message sent to a SOAP API is a request and results in a corresponding response. The response may be valid, or it may be a fault (error) response.
While XML-RPC and SOAP both use XML as the transport language, the current SOAP specification is much more rigid concerning what can and cannot be included in a message.
Each SOAP request includes the following tags:
- XML version (required)
- soap:envelope (required)
- soap:header (optional)
- soap:body (required)
Envelope
is the SOAP-defined tag that wraps header and body. An HTTP request with the Envelope
tag tells anyone who sees it that the interface is using SOAP.
Here's a sample SOAP request (without HTTP headers) for specific account info:
<?xml version = "1.0">
<SOAP-ENV:Envelope
xmlns:SOAP-ENV = "http://www.w3.org/2001/12/soap-envelope"
SOAP-ENV:encodingStyle = "http://www.w3.org/2001/12/soap-encoding">
<SOAP-ENV:Body xmlns:m = "http://www.example.com/">
<m:GetAccount>
<m:AccountID>HVT076236</m:AccountID>
</m:GetAccount>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
Each SOAP response includes the same top level tags as the request. Here's the response to the above request:
<?xml version= "1.0">
<soap:Envelope
xmlns:soap="http://www.w3.org/2003/05/soap-envelope/"
soap:encodingStyle="http://www.w3.org/2003/05/soap-encoding">
<soap:Body>
<m:GetAccountResponse>
<m:Account>Progix Rockets, Inc.</m:Account>
</m:GetAccountResponse>
</soap:Body>
</soap:Envelope>
If, however, the GetAccount procedure were not valid, the system would return a fault response like the following:
<?xml version = '1.0'>
<SOAP-ENV:Envelope
xmlns:SOAP-ENV = "http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsi = "http://www.w3.org/1999/XMLSchema-instance"
xmlns:xsd = "http://www.w3.org/1999/XMLSchema">
<SOAP-ENV:Body>
<SOAP-ENV:Fault>
<faultcode xsi:type = "xsd:string">SOAP-ENV:Client</faultcode>
<faultstring xsi:type = "xsd:string">
Failed to locate method (GetAccount) in class (XYZ) at
/usr/local/abc line 1323.
</faultstring>
</SOAP-ENV:Fault>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
Why SaaS teams and products use SOAP APIs
While the HTTP protocol is sufficient in most cases to support reliability, SOAP APIs take things one step further and allow us to support transactions that comply with ACID (atomicity, consistency, isolation, and durability). ACID is a set of principles that ensure database transactions are processed reliably.
SOAP was built to be extensible. As a result, SOAP APIs can not only support WS-Security, which allows for a greater level of encryption than HTTPS, but many other web services standards, including WS-Addressing and WS-Coordination. In short, SOAP provides developers with an extensive framework beyond the base protocol.
SOAP isn't restricted to a single transfer protocol. Unlike REST (which must use HTTP), SOAP can also use TCP (transmission control protocol), JMS (Java message service), and MQ (message queue), among others. This flexibility gives SOAP an advantage when an integration needs to be based on something other than HTTP. In practice, however, most SOAP APIs are built for HTTP.
SOAP also includes built-in retry logic, meaning teams don't need to add retry logic within the integration itself.
What are best practices for setting up SOAP APIs?
SOAP APIs can be written in just about any language. Make sure your SOAP API:
- Supports only the POST method for HTTP requests.
- Includes a well-defined WSDL (web-service definition) file that matches what the API provides. While it's possible to work with a SOAP API without a WSDL file or with one that doesn't match the API, doing so makes it impossible for devs to programmatically parse the WSDL file to build the SOAP client.
If a SOAP API is too rigid, consider an XML-RPC API instead. It's still based on HTTP with XML as the transport language but lacks much of the complexity that a SOAP API requires.
How do SOAP APIs work with integrations?
As noted above, SOAP allows for more robust security than other APIs (because of the WS-Security extension). You are not required to use WS-Security with SOAP, but it is an excellent reason to select SOAP over REST or another API. As with XML-RPC, you can use regular security features such as API keys and OAuth 2.0 callback URLs with a SOAP API.
Because of its innate complexity, writing code to connect to a SOAP API (that is, writing the SOAP client) is best done by leveraging existing libraries. We can recommend the library for NodeJS (called soap), but there are many others.
Getting a library that allows you to parse the WSDL programmatically (and thereby create a fully functional SOAP client) is the key to making it straightforward to develop against a SOAP API.
For a refresher on SOAP APIs and integrations, check out SOAP APIs: What you Should Know Before You Build A SOAP Integration.
The future of SOAP APIs
SOAP APIs are here to stay even though SOAP has lost popularity due to REST and its close cooperation with HTTP. As newer types of APIs (such as GraphQL) give developers even more options when building APIs, SOAP will probably never return to its former position of prominence for web-based integrations.
That said, SOAP APIs are still found in every corner of the web today, from relatively small and simple implementations to complex ones for massive corporations. When requirements call for a highly dependable and very secure approach to integrations, SOAP APIs are often still the answer.
For more content on SOAP APIs and other integration topics, check out our guide to API integrations.

Want to learn more about API integrations?
Download our API Integrations Guide to see what an API integration is and learn how it works.
Get my CopyAbout Prismatic
Prismatic, the world's most versatile embedded iPaaS, helps B2B SaaS teams launch powerful product integrations up to 8x faster. The industry-leading platform provides a comprehensive toolset so teams can build integrations fast, deploy and support them at scale, and embed them in their products so customers can self-serve. It encompasses both low-code and code-native building experiences, pre-built app connectors, deployment and support tooling, and an embedded integration marketplace. From startups to Fortune 100, B2B SaaS companies across a wide range of verticals and many countries rely on Prismatic to power their integrations.