Synchronous and Asynchronous Invocations
Integrations are configured by default to run asynchronously. That means that whenever an integration is invoked by trigger webhook URL, the integration begins to run and the system that invoked the integration can go on to complete other work. This is the most common case for integrations - you want to start up an instance when some certain event occurs, but you don't want to wait around while the instance runs.
Sometimes, though, it's handy for an application to get information back from the instance that was invoked. For example, you might want your proprietary software to wait until an instance runs to completion before completing other work. In that case, you can choose to run your integration synchronously. Then, when your software makes a call to the instance's webhook trigger URL the HTTP request is held open until the instance run is complete.
When you choose to run your integrations synchronously, the HTTP request that invokes an instance returns a redirect to a URL containing the output results of the final step of the integration. For example, if the final step of your integration pulls down JSON from https://jsonplaceholder.typicode.com/users/1, you will see this when you invoke the integration synchronously:
curl 'https://hooks.prismatic.io/trigger/EXAMPLE==' \
--data '{}' \
--header "Content-Type: application/json" \
--header "prismatic-synchronous: true" \
--location
{"id":1,"name":"Leanne Graham","username":"Bret","email":"Sincere@april.biz","address":{"street":"Kulas Light","suite":"Apt. 556","city":"Gwenborough","zipcode":"92998-3874","geo":{"lat":"-37.3159","lng":"81.1496"}},"phone":"1-770-736-8031 x56442","website":"hildegard.org","company":{"name":"Romaguera-Crona","catchPhrase":"Multi-layered client-server neural-net","bs":"harness real-time e-markets"}}
You can toggle if your integration is synchronous or asynchronous by clicking the trigger and selecting a Response Type.
You can also pass in a header, prismatic-synchronous
with a webhook invocation to instruct your instance to run synchronously or asynchronously:
curl 'https://hooks.prismatic.io/trigger/EXAMPLE==' \
--header "prismatic-synchronous: false" \
--request POST
Synchronous invocations and redirects
When you invoke an instance synchronously, the HTTP response you receive contains the result of the final step of your flow.
If the result is over 5MB in size, the result is written to Amazon S3, and you receive an HTTP 303 redirect to an object in S3.
Because of this possible redirect, you should ensure that your HTTP client is configured to follow HTTP status code 303 redirects.
For curl
, for example, include a -L / --location
flag so it follows redirects.
curl 'https://hooks.prismatic.io/trigger/EXAMPLE==' \
--header "prismatic-synchronous: true" \
--location \
--request POST \
--data "{}"
If you would like Prismatic's runner to always return 303 redirects, you can include an optional header, prismatic-prefer-redirect-sync-response: true
and the runner will return an HTTP 303 response to an S3 object, regardless of size.
You can control the content-type
of the response by adding a contentType
property to your last step's result.
For example,
const returnData = `<note>
<to>Tove</to>
<from>Jani</from>
<heading>Reminder</heading>
<body>Don't forget me this weekend!</body>
</note>`;
return {
data: returnData,
contentType: "application/xml",
};
HTTP status codes for synchronous integrations
When an instance is configured to run synchronously or is invoked synchronously with the prismatic-synchronous
header, the HTTP response returns a status code 200 - OK
by default.
It's sometimes useful, though, to return other HTTP status codes.
For example, if a client submits wrongly formatted data to be processed by an instance, it might be helpful to return a 406 - Not Acceptible
or 415 - Unsupported Media Type
.
- Low-Code
- Code-Native
To accomplish this in a low-code integration, you can configure the final step of your integration to return a different status code. Most commonly, you can add a Stop Execution step to the end of your integration, and specify an HTTP response that it should return.
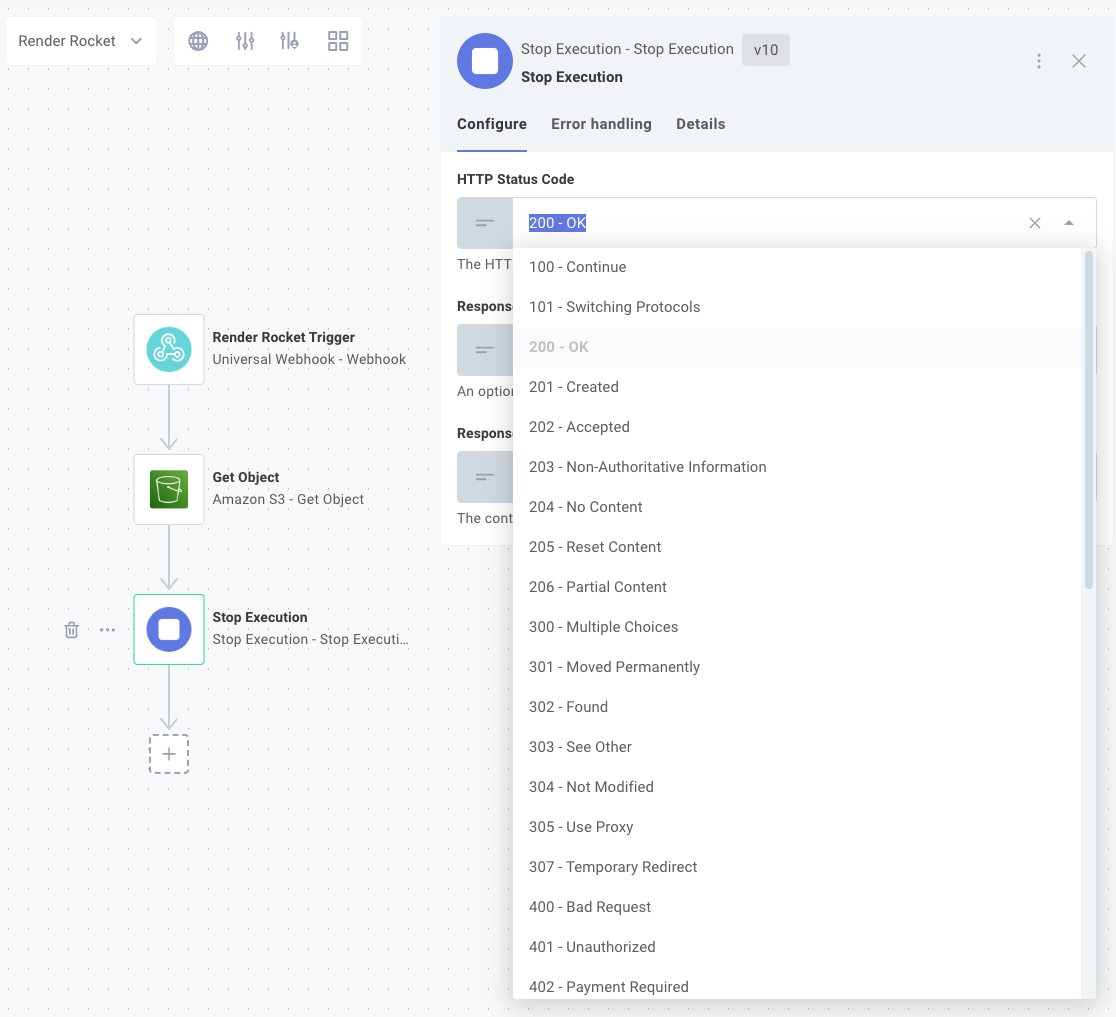
If you would like to return HTTP status codes from a custom component at the end of your integration instead, return an object with a statusCode
attribute instead of a data
attribute:
return { statusCode: 415 };
To accomplish this in a code-native integration, mark your flow as isSynchronous: true,
and return an object with a statusCode
attribute:
export const flow1 = flow({
name: "Flow 1",
stableKey: "9499d1d8-dddd-4d9b-aaff-c054f59d02cc",
description: "This is the first flow",
isSynchronous: true,
onExecution: async (context, params) => {
return {
data: {
customError: "Invalid file type. You must provide an SVG.",
},
statusCode: 415,
headers: { "X-Custom-Header": "foo" },
contentType: "application/json",
};
},
});
$ curl 'https://hooks.prismatic.io/trigger/EXAMPLE==' \
--verbose \
--location \
--header "prismatic-synchronous: true"
* TCP_NODELAY set
* Connected to hooks.prismatic.io (13.227.37.2) port 443 (#0)
...
< HTTP/2 415
Response headers for synchronous integrations
In addition to HTTP status codes (above), you can also yield custom response headers from your synchronous integrations. This is handy if you would like to redirect the client to a different URL once the flow is complete.
Your code step, for example, can read:
module.exports = async ({ logger, configVars }, stepResults) => {
return {
data: "Redirecting you...",
statusCode: 303,
headers: { Location: "https://example.com" },
};
};
When a client invokes the integration synchronously, they will receive a 303 - See Other
status code and be redirected to https://example.com
.
Synchronous call limitations
Response body and status code limitations
When an integration is invoked synchronously, the integration redirects the caller to a URL containing the output results of the final step of the integration.
If the final step of the integration is a Stop Execution action, or any custom component action that returns a statusCode
, the redirect does not occur and the caller receives a null
response body instead.
API gateway size and time limitations
AWS API Gateway times out requests after 29 seconds, and our maximum response size is 500MB. So, to get a response from an instance that is invoked synchronously, please ensure that your integration runs in under 29 seconds and produces a final step payload of less that 500MB.
If your integration regularly takes over 29 seconds to run, or produces large responses, we recommend that you run your integrations asynchronously instead.
When you invoke an integration asynchronously you receive an executionId
:
curl 'https://hooks.prismatic.io/trigger/EXAMPLE==' \
--data '{}' \
--header "Content-Type: application/json"
{"executionId":"SW5zdGFuY2VFeGVjdXRpb25SZXN1bHQ6OTdiNWQxYmEtZGUyZi00ZDY4LWIyMTgtMDFlZGMwMTQxNTM5"}
That execution ID can be exchanged later with the Prismatic API for logs and step results using the executionResult GraphQL mutation.