Looping
For many integrations it's handy to be able to loop over an array of items, or to loop a certain number of times. If your integration processes files on an SFTP server, for example, you might want to loop over an array of files on the server. If your integration sends alerts to users, you might want to loop over an array of users.
Prismatic provides the loop component to allow you to loop over an array of items, or you can loop a predetermined number of times. After adding a loop step to your integration, you can then add steps within the loop that will execute over and over again.
The loop components takes one input: a items. Items is an array - an array of numbers, strings, objects, etc. For example, one step might generate an array of files that your integration needs to process. Its output might look like this:
[
"path/to/file1.txt",
"path/to/file2.txt",
"path/to/file3.txt",
"path/to/file4.txt"
]
The loop component can then be configured to loop over those files by referencing the results
of the list files step:
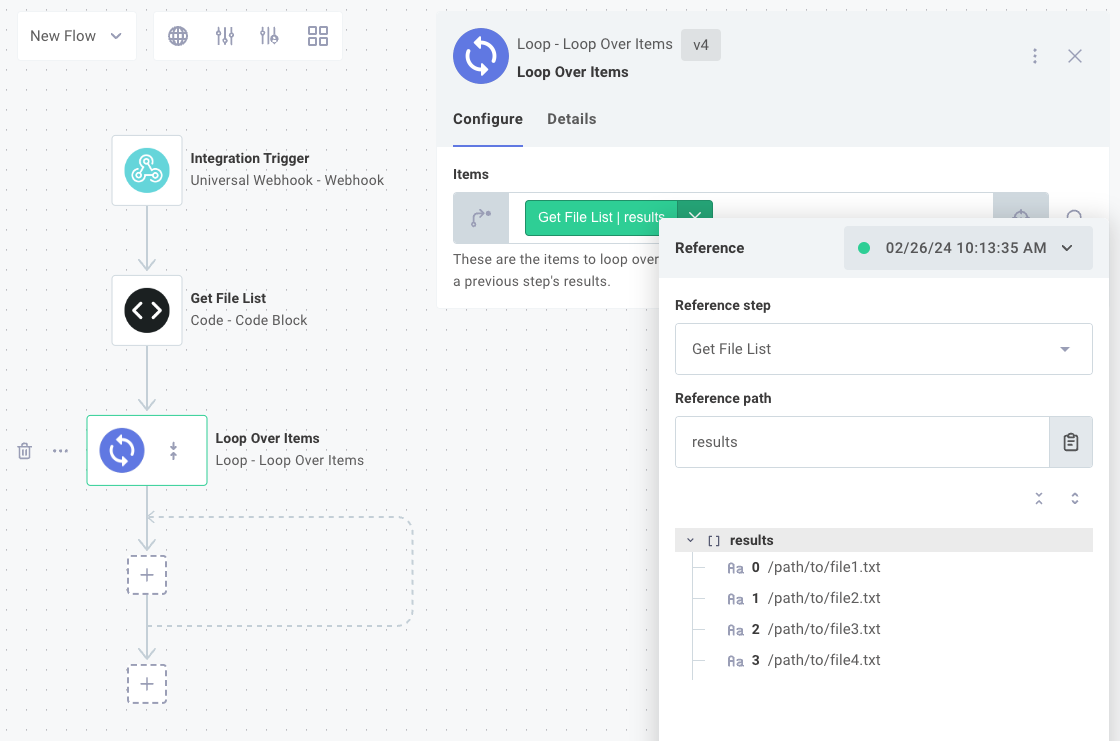
Subsequent steps can reference the loop step's currentItem
and index
parameters to get values like path/to/file3.txt
and 2
respectively:
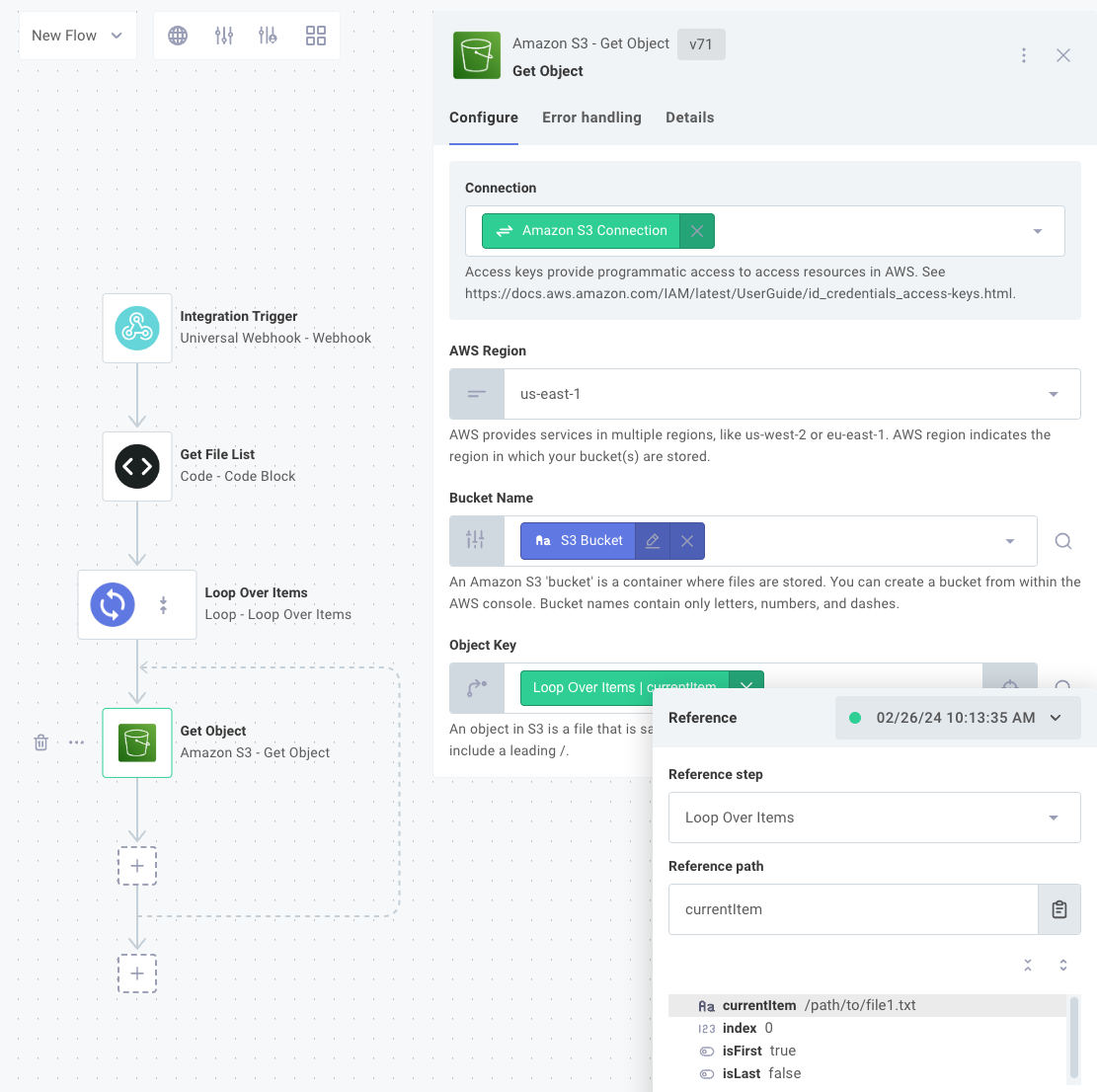
For More Information: The Loop Component, Looping Over Files Quickstart, Looping Over a Paginated API
Looping over lists of objects
The list of objects passed into a loop component can be as simple or complex as you like.
In this example, if we have a loop named Loop Over Users, and the loop was presented items in the form:
[
{
"name": "Bob Smith",
"email": "bob.smith@progix.io"
},
{
"name": "Sally Smith",
"email": "sally.smith@progix.io"
}
]
Then the loop will iterate twice - once for each object in the list, and we can write a code component that accesses the loop's currentItem
and index
values and sub-properties of currentItem
like this:
module.exports = async (
{ logger },
{ loopOverUsers: { currentItem, index } },
) => {
logger.info(`User #${index + 1}: ${currentItem.name} - ${currentItem.email}`);
};
That will log lines like User #1: Bob Smith - bob.smith@prismatic.io
.
Looping over a paginated API
Many third-party APIs limit the number of records you can fetch at once, and let you load a batch (or "page") of records at a time. You may need to loop over an unknown number of pages of records in an integration.
You can accomplish that with a combination of two loops (one to loop over pages, and one to loop over records on each page) and a break loop action that stops loading pages when there are no more left to load:
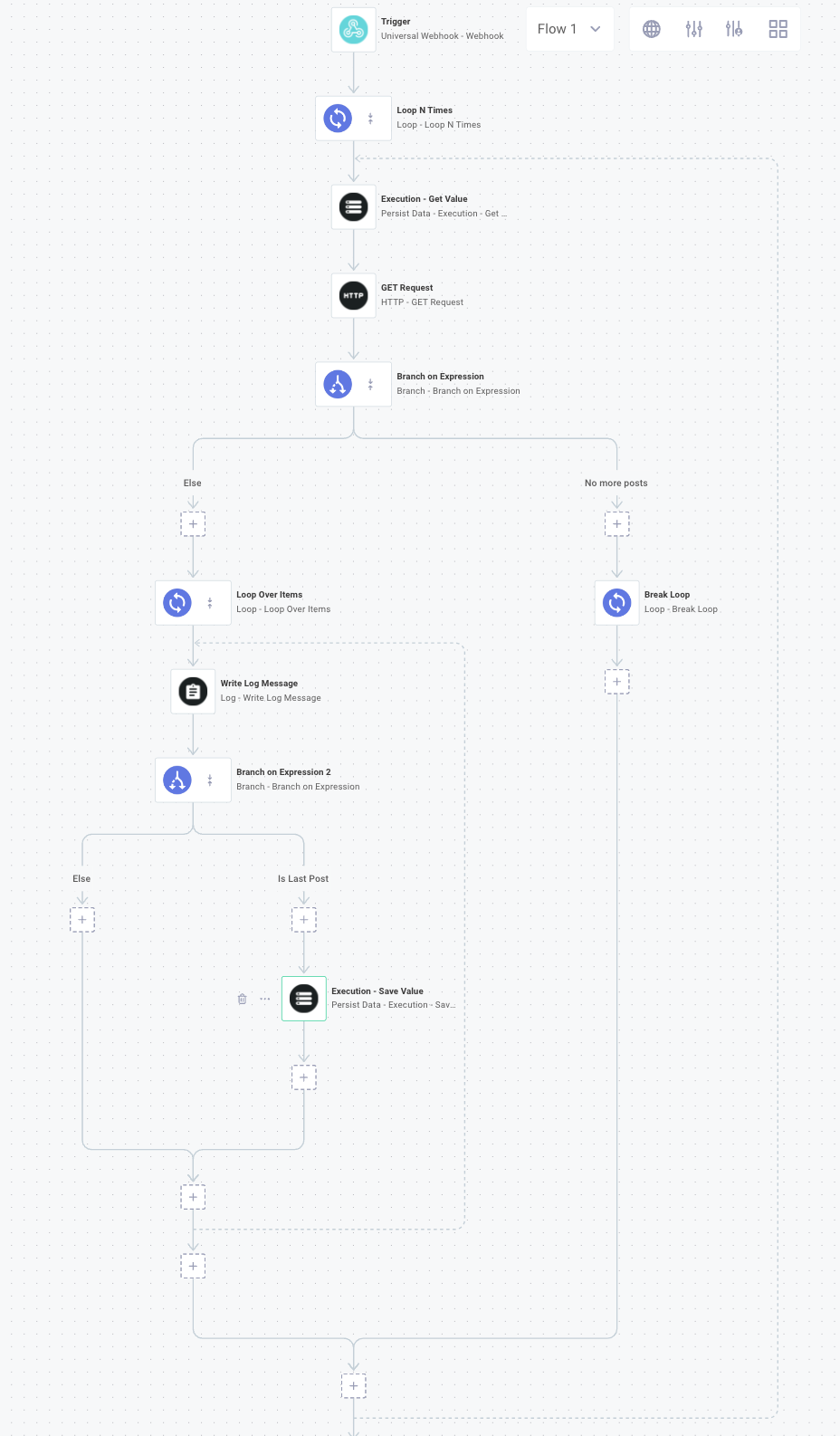
Please reference this quickstart for an example of how to loop over a paginated API.
Return values of loops
A loop will collect the results of the last step within the loop, and will save those results as an array. For example, if the loop is presented the list of JSON-formatted user objects above, and the last step in the loop is a code component reading:
module.exports = async(context, loopOverUsers: { currentItem }) => {
return {data: `Processed ${currentItem.email}`}
}
Then the result
of the loop will yield:
["Processed bob.smith@progix.io", "Processed sally.smith@progix.io"]