Translations and Internationalization (i18n)
Translations and internationalization
Your customers may not speak English. Internationalization (i18n) allows you to translate the embedded marketplace into other languages.
Examine marketplace's translations package. This repo contains all phrases in marketplace that you can override, along with their English translations. There are three types of phrases that can to be translated:
SimplePhrase
phrases are direct string-to-string translations. For example,input.integrationVersionLabel
"Integration Version" may translate to French "Version d'intégration".ComplexPhrase
phrases are templated strings, where a set of variables representing customer name, integration name, counts, etc., will be templated into the string. For example,integrations.id__banner.customerActivateText
could have a value of"Please contact %{organization} to activate this integration."
This will template your organization's name into the string.- Dynamic Phrases represent translations of your content (like integration name, config variable key, etc), and is described below.
An example of i18n in action is available in our example embedded app on GitHub.
Adding an i18n dictionary for a user
To apply an i18n dictionary for a user, add a translation
property to your prismatic.init
invocation.
Phrases can also be added to prismatic.showMarketplace
or similar functions to apply translations to just a specific iframe.
Include any phrases
that you would like to translate:
prismatic.init({
translation: {
phrases: {
// Static Translations:
"integration-marketplace__filterBar.allButton": "Alle, bitte!",
"integration-marketplace__filterBar.activateButton": "Solo activado",
"detail.categoryLabel": "カテゴリー",
"detail.descriptionLabel": "विवरण",
"detail.overviewLabel": "概述",
// Complex translation with variables:
"activateIntegrationDialog.banner.text--isNotConfigurable": {
_: "Veuillez contacter %{organization} pour activer cette intégration",
},
},
},
});
After making changes, reload marketplace. You will see the new translations.
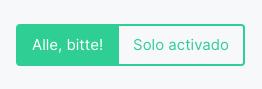
The Marketplace NPM package includes helpful comments that show current English values for phrases you can translate. Enable intellisense in your code editor and mouse over the phrase you want to translate to see the English version of the phrase.
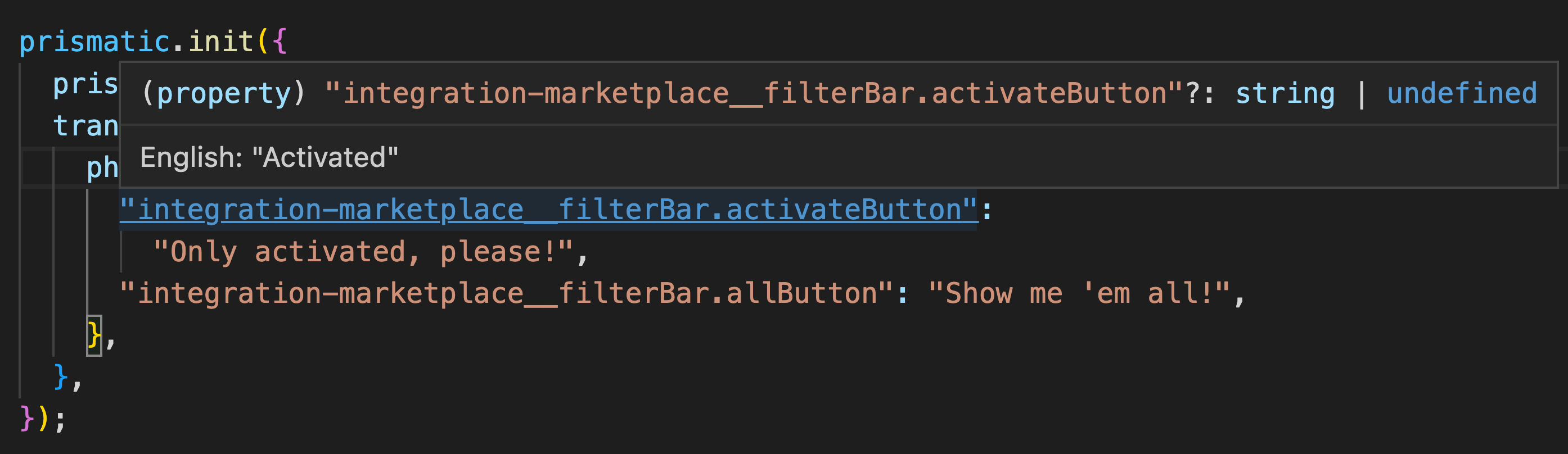
i18n debug mode
If you would like to identify what phrase matches what UI element in Prismatic, turn on debug mode by adding debugMode: true
to the translation
property of prismatic.init
:
prismatic.init({
translation: {
debugMode: true,
},
});
This will cause all UI elements in an embedded marketplace to show their phrase's key names and current values:
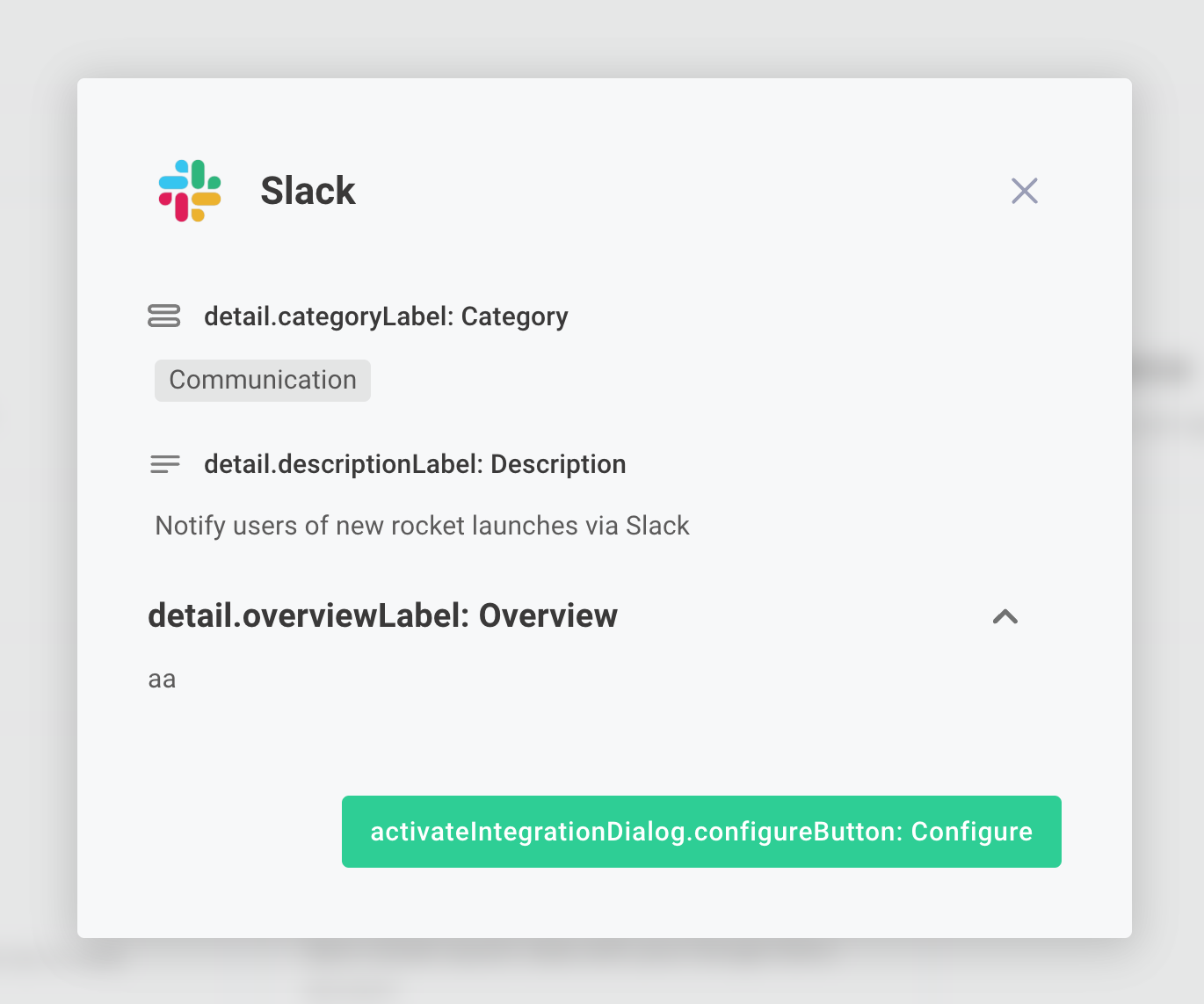
Namespaced phrases
Some phrases are shared across several pages in the embedded marketplace. A list of these common/shared phrases are available on GitHub.
For example, common.loading
translates to "Loading"
in English on several screens.
You can set common.loading
once, and that translation will affect multiple screens.
If you would like to customize common phrases on a per-page basis, you can namespace phrases.
For example, if you would like to translate common.loading
to a different phrase, but only on the alert monitors page, prepend the phrase key with a PhraseNamespace
, entering NAMESPACE__PHRASE-KEY
(see translations):
prismatic.init({
translation: {
phrases: {
"integrations.id.alert-monitors__common.loading": "Sit tight a sec...",
},
},
});
Dynamic Phrases
As you build integrations you create several phrases that are unique to your organization. These unique phrases include:
- Integration name
- Config variable name
- Config wizard page title
- Config wizard page description
- Config wizard helper text
- Flow names (customers see these if they look at execution results pages)
- Step names (customers see these if they look at execution results pages)
You can translate these phrases by adding a dynamicPhrase
property to the phrases
object.
For example, if your integration is named "Sync customer data with Salesforce"
and you would like to translate it to French, add a dynamicPhrase
to your prismatic.init
invocation:
prismatic.init({
translation: {
phrases: {
dynamicPhrase: {
"Sync customer data with Salesforce":
"Synchronisez les données clients avec Salesforce",
},
},
},
});
You can override any other dynamic phrases in the same way.
prismatic.init({
translation: {
phrases: {
dynamicPhrase: {
"Microsoft Teams": "Microsoft Squadre", // Integration name
"Notify a Teams channel of new leads":
"Notifica un canale di Teams di nuovi lead", // Integration description
"Teams Configuration": "Configurazione di Teams", // Config wizard page title
"Enter your Teams authentication info": "", // Config wizard page subtitle
"Teams Authentication": "Autenticazione di Teams", // Config variable
"<h1>Teams OAuth</h1>": "<h1>OAuth di Teams</h1>", // HTML helper text in the config wizard
},
},
},
});
Dynamic phrases must match the exact phrase that you use in your integration.
If your config variable has a capital letter or punctuation, you must include that in the dynamic phrase.
Partial matches will not translate (for example, if you translate {"Customer": "Cliente"}
, a config variable named "Customer Name"
will not translate to "Cliente Name"
).
Note the <h1>
portion of the last example.
If you provide your own HTML helper text in your config wizard, you must translate the full HTML string.
Listing all dynamic phrases
If you would like to see a list of all dynamic phrases that you can translate from your account, you can do so using prism
.
prism translations:list
A file called translations_output.json
will be created in your current directory containing all dynamic phrases for your account or for a specific integration - you can use that file as a reference as you fill out your dynamicPhrase
object.