Managing Customers
Creating new customers
- Web App
- CLI
- API
After clicking on the Customers link on the left-hand sidebar, click the + Add Customer button on the upper-right. Enter an appropriate name and description for your customer.
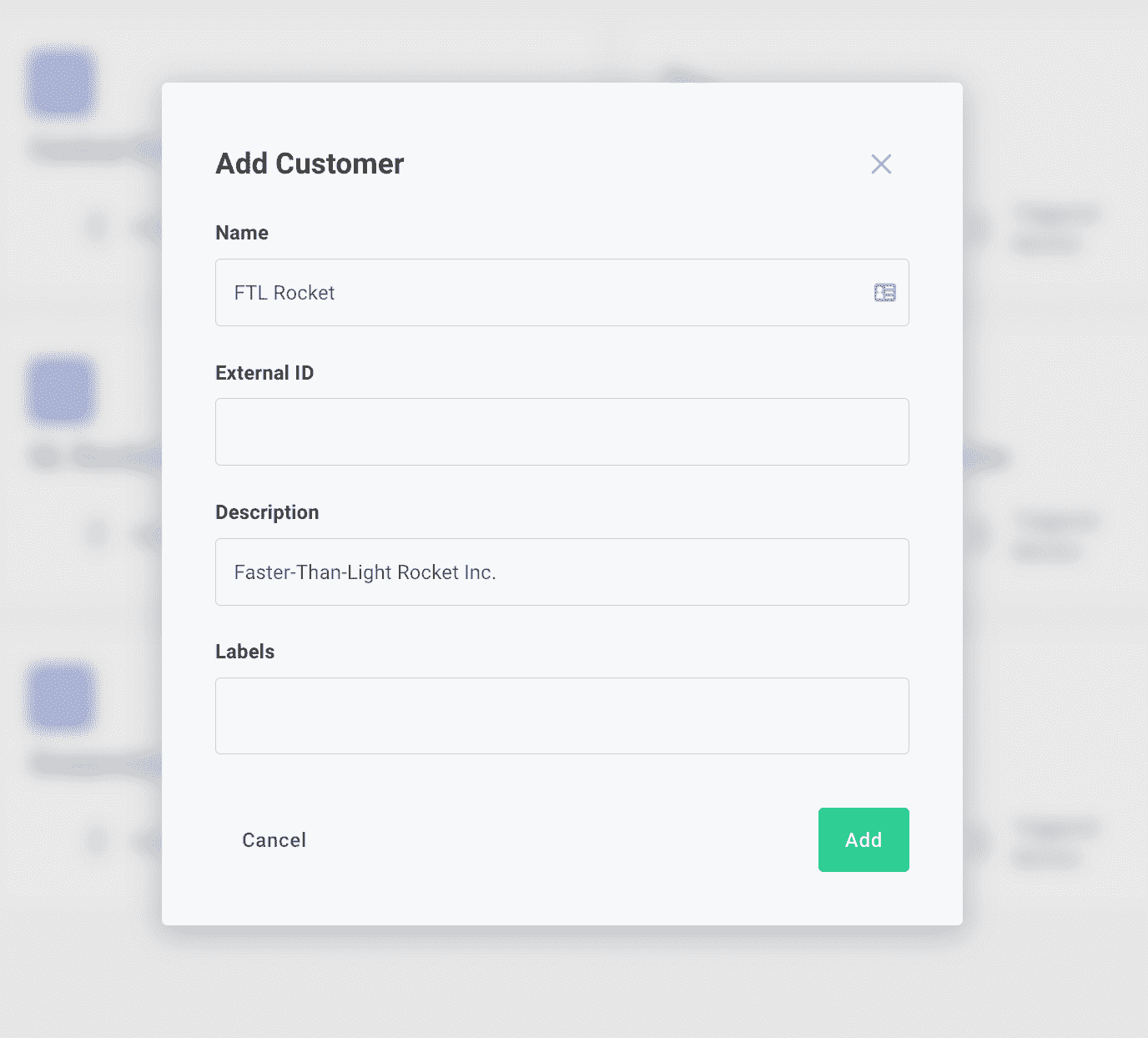
To create new customers, use the prism customers:create
subcommand.
prism customers:create \
--name 'FTL Rockets' \
--description 'Faster-Than-Light Rocket Inc'
Create a new customer using the createCustomer mutation:
mutation {
createCustomer(
input: { name: "FTL Rockets", description: "Faster-Than-Light Rocket Inc" }
) {
customer {
id
}
}
}
If you are using embedded to present an integration marketplace or workflow builder in your app, you must create customers that have external IDs (you can do this through the Web App, CLI or API), but you should not create customer users.
Customer users are automatically created for you when you sign a JWT for authentication. Embedded customer users are different from standard customer users - standard customer users require a valid email address and receive Prismatic-branded confirmation emails. Embedded customer users can have any value (like a UUID) for their unique identifier and do not receive transactional emails.
Searching customers
- Web App
- CLI
- API
After clicking the Customers link on the left-hand sidebar, you can enter a portion of a customer's name into the search bar to filter customers by name. To filter customers by description, external ID, or label instead, click the Filter button to the right of the search bar.
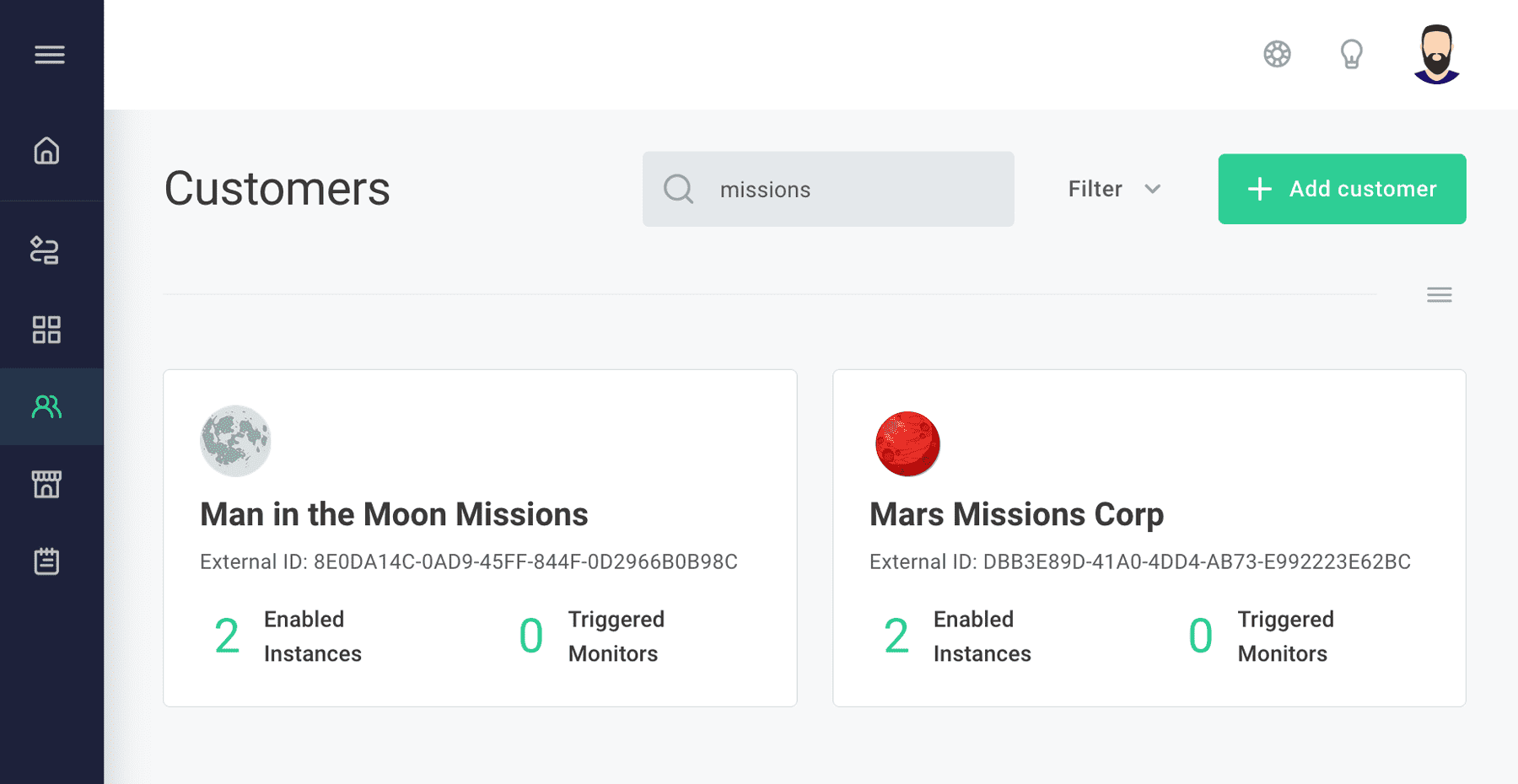
You can list customers using prism customers:list
, and can --filter
or --sort
the results:
prism customers:list --filter "Name=Smith Rocket Company"
Query customers for a list of customers:
query {
customers {
nodes {
id
name
description
}
}
}
Modifying customers
After clicking the Customers link on the left-hand sidebar, you will be presented with a list of your customers.
Clicking the name of any customer will bring you to the customer's page. This page contains a menu with options to manage instances assigned to the customer, alert monitors, logs, customer users, and file attachments.
Editing customer name, description and logo
- Web App
- CLI
- API
From the customer's page, click into the Details tab on the top of the page to change the customer's name or modify the longer customer description. To modify the customer's avatar icon, click the Upload a photo link on the Details tab. The avatar icon you upload will be resized and cropped to 500 x 500 pixels. Transparent PNG images tend to look the best.
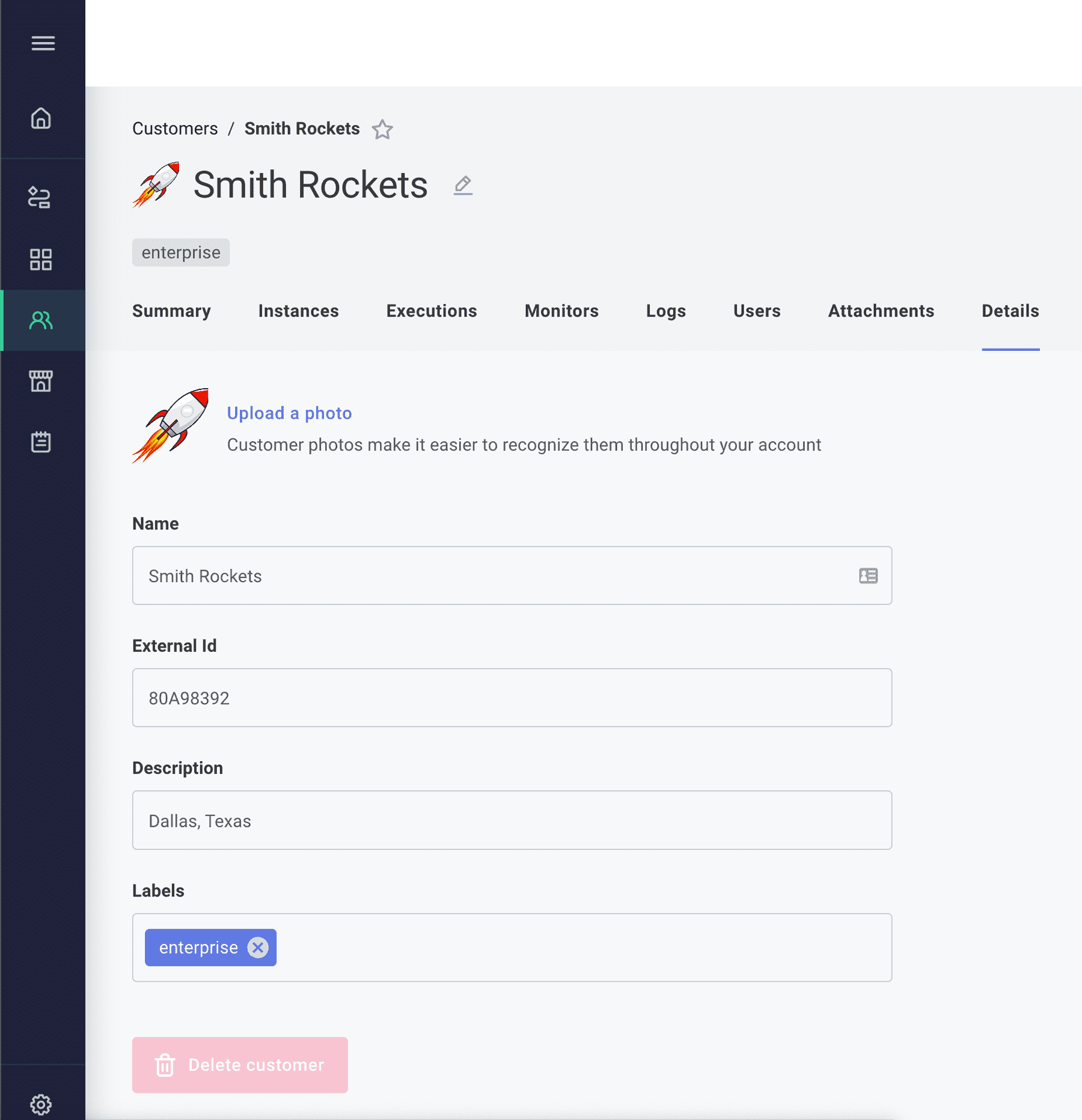
Update the customer name or description from the command line using the prism customers:update
subcommand with the customer's ID:
prism customers:update \
EXAMPLEtZXI6M2JkYzcwNTAtZTU2ZS00ZGJkLThmMzQtNWI0MDdhOTEXAMPLE \
--name "New Customer Name" \
--description "New Customer Description"
Use the updateCustomer mutation to update a customer:
mutation {
updateCustomer(
input: {
id: "Q3VzdG9tZXI6ZDIyOGUwNjItYzc0NC00NDFkLWE0MDMtNjQ1NTU4MDQ1OTZk"
name: "New Customer Name"
description: "New customer description"
}
) {
customer {
id
}
}
}
Customer labels
Labels help you keep your customers organized. You can assign any number of labels to a customer from the customer's summary tab, and can then search for customers by label. Note: for consistency, labels are always lower-cased.
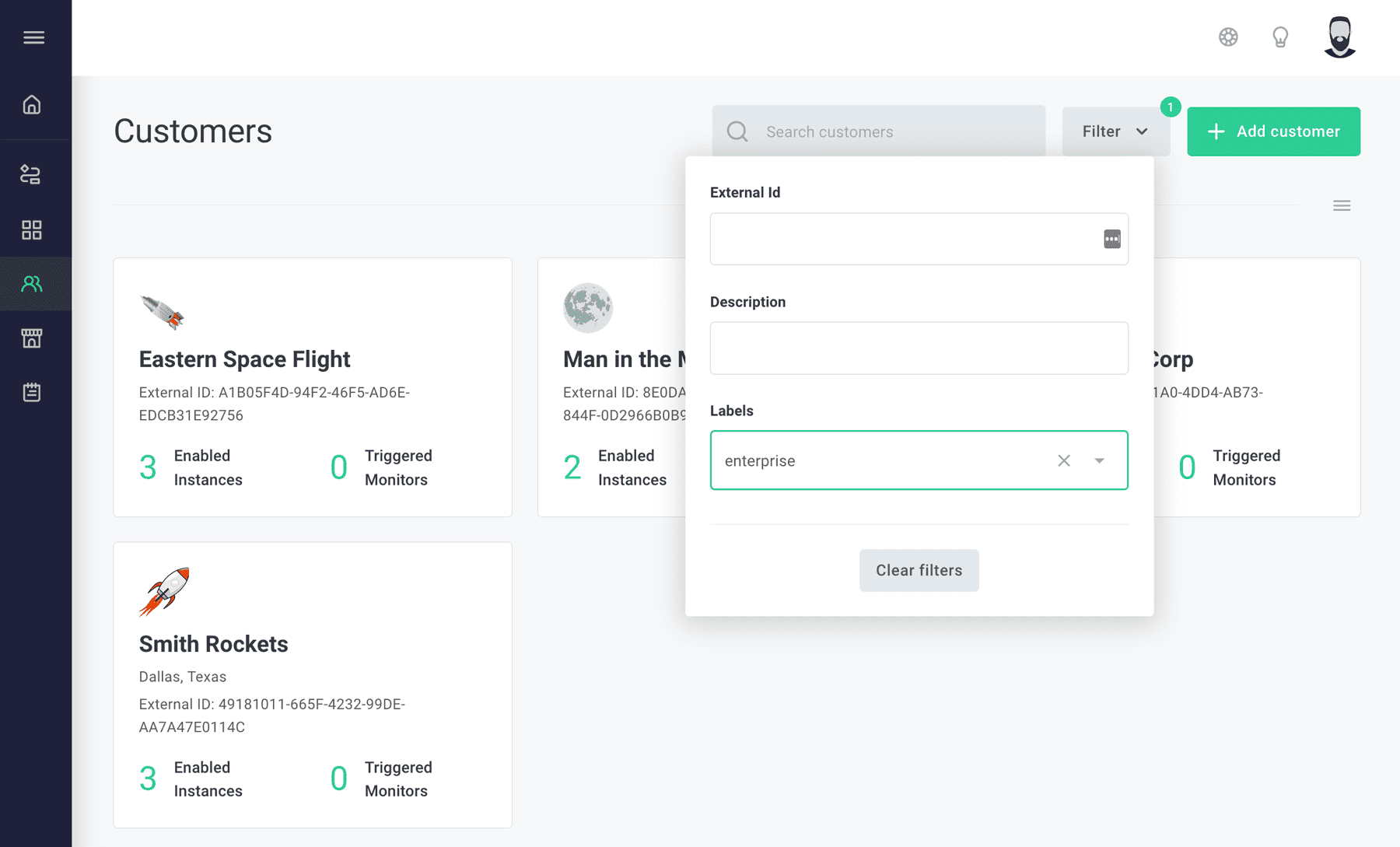
Deleting customers
When a customer is deleted, all associated users, and instances are also deleted. Use caution when deleting customers.
- Web App
- CLI
- API
After clicking on the Customers link on the left-hand sidebar, click the name of the customer you would like to delete, and then select the Details tab.
Verify that the name shown matches the customer you wish to delete, and click the Delete customer button. Confirm your choice by typing the customer name exactly, and then click Remove customer.
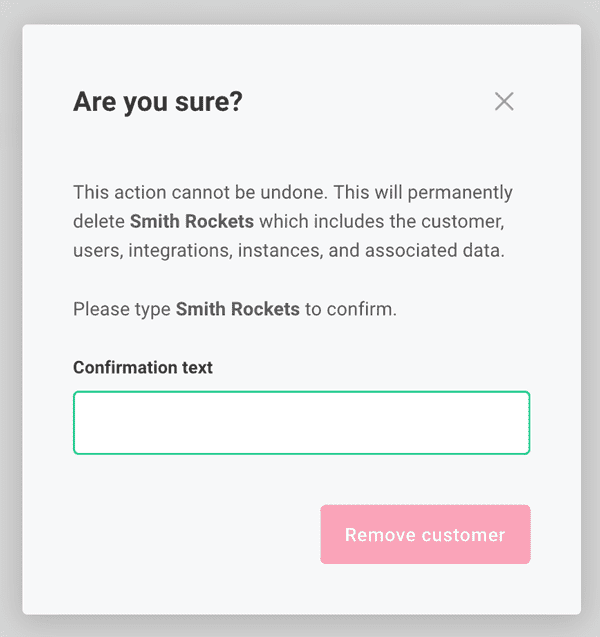
To delete a customer, use the prism customers:delete
subcommand.
# Get the customer's ID
CUSTOMER_ID=$(prism customers:list \
--columns id \
--filter 'Name=^FTL Rockets$' \
--no-header)
prism customers:delete ${CUSTOMER_ID}
Delete a customer using the deleteCustomer mutation:
mutation {
deleteCustomer(
input: {
id: "Q3VzdG9tZXI6ZDIyOGUwNjItYzc0NC00NDFkLWE0MDMtNjQ1NTU4MDQ1OTZk"
}
) {
customer {
id
}
}
}
Customer external IDs
A customer can be assigned an External ID - a unique identifier from an external system.
So, if you know "Smith Rocket Company" as a customer with an ID of abc-123
in another system you use, you can assign "Smith Rocket Company" in Prismatic the externalId
of abc-123
.
This is helpful if you need to quickly look up or associate customers in Prismatic with customers in your external system.
External IDs can be set programmatically (see the next section), or can be edited within the Prismatic we app by clicking the Customers link on the left-hand sidebar, selecting a customer, and then clicking on the Details tab.
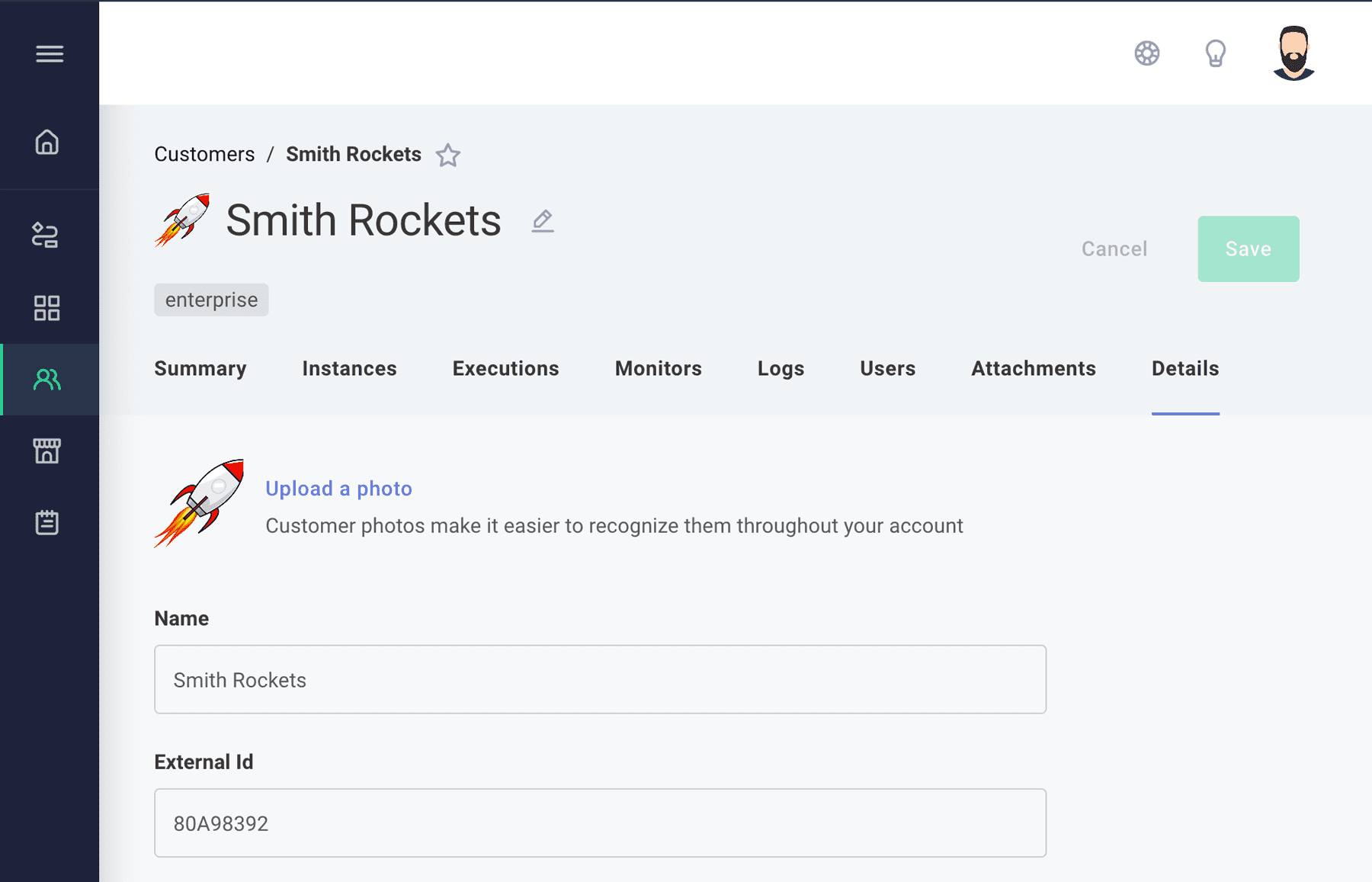
External IDs are required if you want to route webhook requests to instances deployed to specific customers using shared webhook triggers.