Text Manipulation Component

Perform common operations on strings and arrays of strings
Component key: text-manipulation
Description
The text manipulation component allows you to perform common operations on some text. For example, you can join two or more text strings together with the join action, find text with the match action, replace strings with other strings within a piece of text with the replace function, and more.
Actions
Decode Base64
Convert the input string from base64 encoding | key: decodeBase64
Input | Notes | Example |
---|---|---|
Base64 Text string / Required text | SGVsbG8sIFByaXNtYXRpYyE= |
{
"data": "Example"
}
Encode Base64
Convert the input string or file to base64 encoding | key: encodeBase64
Input | Notes | Example |
---|---|---|
Text or file string / Required text | Hello, world; how are you? |
{
"data": "SGVsbG8sIFByaXNtYXRpYyE="
}
Extract Substring
Extract a substring from a string | key: slice
Input | Notes | Example |
---|---|---|
Slice start string / Required sliceStart | The index on which to start the slice of the text. | 5 |
Slice stop string sliceStop | The index on which to stop the slice of the text. | 7 |
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
This action uses the JavaScript slice method to extract substrings from a string.
{
"data": "Example"
}
Find & Replace
Find and replace all instances of one substring with another | key: replace
Input | Notes | Example |
---|---|---|
Substring to find and be replaced string / Required find | This is the substring that is to be replaced. | Hi |
The substring to replace instances of 'find' with string replace | The substring to replace instances of 'find' with. Can be a string or regular expression. | Hello |
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
This action uses the JavaScript replace method and a regex global flag to replace all instances of find with replace. Find can be a regular expression or a string.
{
"data": "Example"
}
Find & Replace Multiple Substrings
Find and replace all instances of multiple substrings with another | key: replaceMultipleSubstring
Input | Notes | Example |
---|---|---|
Replace With string / Required Key Value List replaceMultiple | Replace | |
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
{
"data": "Example"
}
HTML Decode
Decode HTML-encoded input string | key: htmlDecode
Input | Notes | Example |
---|---|---|
Text string / Required text | This is the text to manipulate | foo © bar ≠ baz 𝌆 qux |
{
"data": "foo © bar ≠ baz 𝌆 qux"
}
HTML Encode
Convert input string to HTML-encoded equivalent | key: htmlEncode
Input | Notes | Example |
---|---|---|
Text string / Required text | This is the text to manipulate | foo © bar ≠ baz 𝌆 qux |
{
"data": "foo © bar ≠ baz 𝌆 qux"
}
Join
Join strings together using an optional separator to form a single string. | key: join
Input | Notes | Example |
---|---|---|
Separator string separator | The separator to use to split or concatenate text. | / |
Strings string Value List strings | A set of strings to join together into a single string. |
This action is helpful when you want to generate messages, file paths, etc., based off of results from several steps.
As an example, suppose you would like to generate a file path of the form:
${bucketName}://${customerName}/items/${itemNumber}.txt
Where bucketName
and customerName
are config variables, and itemNumber
is an output from a previous step.
You can reference the config variables, and step output, and place string literals (://
, etc) between them:
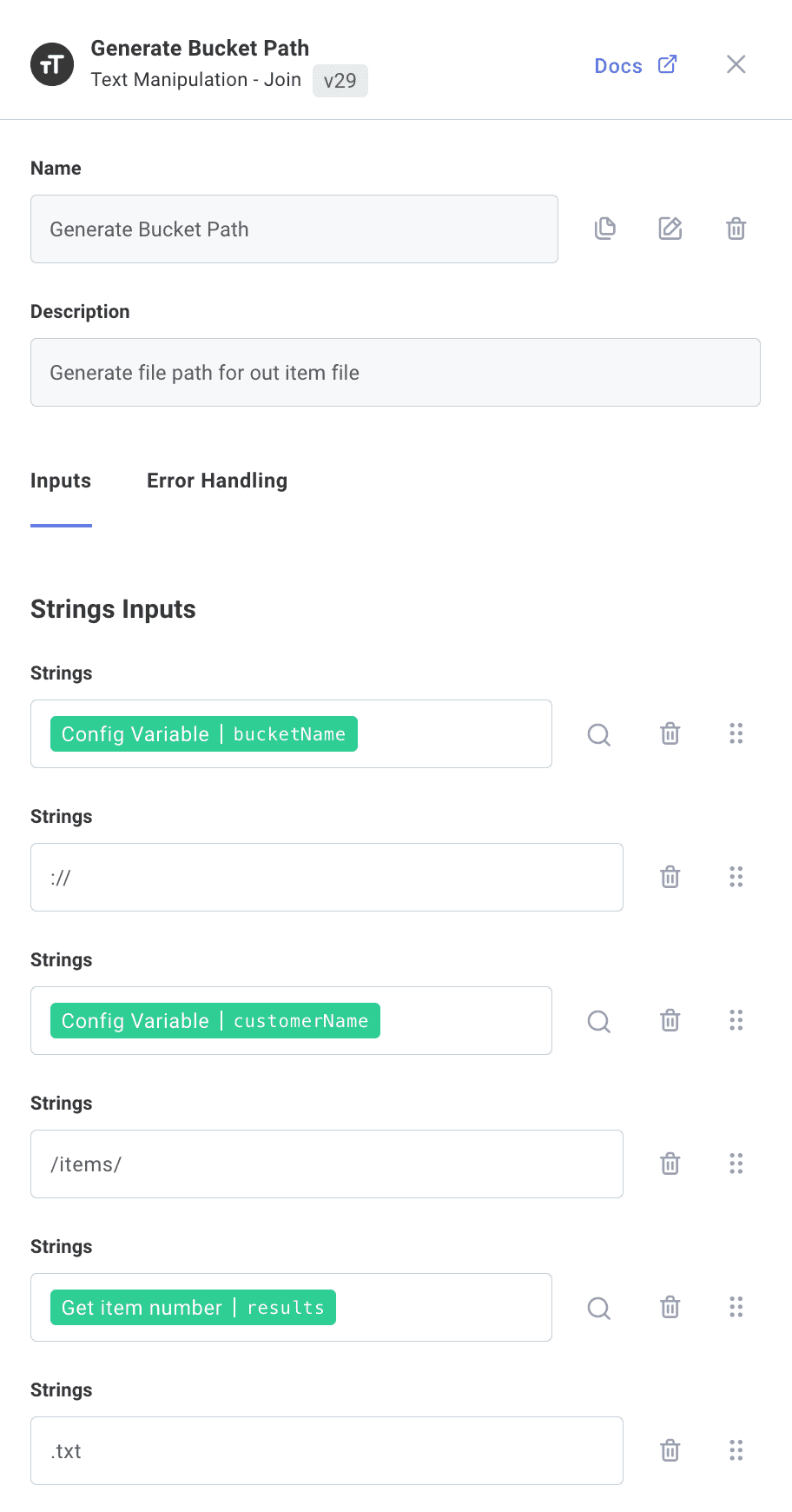
String concatenation can be done from within an input using template inputs
{
"data": "Example"
}
Join Lines
Join strings together with a newline character | key: joinLines
Input | Default | Notes | Example |
---|---|---|---|
Newline type string / Required newlineType | The type of newline to use. | ||
Number of newlines string / Required numberOfNewlines | 1 | The number of newlines to insert. | 2 |
Strings string Value List strings | A set of strings to join together into a single string. |
The Join Lines action is used to join strings together with one or multiple newline characters. This action is particularly useful when you need to combine multiple lines of text into a single text block with line breaks.
As an example, suppose you're building an integration workflow for a data export task. You have a list of data records, and you want to format these records into a CSV (Comma-Separated Values) string for easy export. Each data record is represented as a string, and you need to concatenate them with commas as column separators and newline characters as row separators.
["John,Doe,30","Alice,Smith,25","Bob,Johnson,35"]
Now, you want to create a CSV string from these data records. You use the "Join Lines" action as follows:
Use the Strings input to add the lines. Example: John,Doe,30. Use the Newline type input to set the type of newline to use, LF for Unix systems or CRLF for Windows systems. Use the Number of newlines to add the number of newlines between each line of strings.
Example output using 1 newline:
John,Doe,30
Alice,Smith,25
Bob,Johnson,35
{
"data": "Line1\nLine2"
}
Lower Case
Convert the input string to lower case | key: lowerCase
Input | Notes | Example |
---|---|---|
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
{
"data": "example"
}
Match Regex
Match a string against a regular expression | key: match
Input | Default | Notes | Example |
---|---|---|---|
Regex string / Required regex | A regular expression to match against the text that is supplied. | ^[A-Z0-9._%+-]{1,64}@(?:[A-Z0-9-]{1,63}\.){1,125}[A-Z]{2,63}$ | |
Regex Flags string regexFlags | g | Flags to apply to the regular expression. For example, you can specify 'd' to ensure capture groups are returned. See https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_expressions#advanced_searching_with_flags | |
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
This action applies the Javascript match method to the text you input with a regex global flag.
For example, if you enter The quick brown fox jumps over the lazy dog. It barked.
as your text input, and [A-Z]
as your regular expression, this action will return the list ["T","I"]
.
{
"data": [
"Example Match 1",
"Example Match 2"
]
}
Remove Whitespace
Remove leading and trailing whitespace from a string | key: trim
Input | Notes | Example |
---|---|---|
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
This action will remove leading and trailing whitespace, converting something like " This is a test! "
to "This is a test!"
.
{
"data": "Example"
}
Split Lines
Split a block of text on newline characters (\n) | key: splitLines
Input | Default | Notes | Example |
---|---|---|---|
Filter Blank Lines boolean filterBlankLines | true | Filter blank lines (like trailing newlines) | |
Text string / Required text | This is the text to manipulate | My first line My second line My third line |
{
"data": [
"My first line",
"My second line",
"My third line"
]
}
Split String
Split a string into a list of strings on a separator character | key: split
Input | Notes | Example |
---|---|---|
Separator string separator | The separator to use to split or concatenate text. | / |
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
This action is useful to split a string into a list of strings.
For example, given a string /usr/local/lib/python3
and separator /
, this action produces ["usr","local","lib","python3"]
.
{
"data": [
"Example Part 1",
"Example Part 2"
]
}
Upper Case
Convert the input string to UPPER CASE | key: upperCase
Input | Notes | Example |
---|---|---|
Text string / Required text | This is the text to manipulate | Hello, world; how are you? |
{
"data": "EXAMPLE"
}