Change Data Format Component

Change data from one format to another
Component key: change-data-format
Description
The change data format component allows you to convert data between common formats, like JSON, XML, CSV, and YAML, and to serialize (turn an object into a string) or deserialize (turn a string into an object) each supported format.
For example, the "JSON to XML" action will convert a JSON string that looks like this:
{
"user": {
"name": "John Doe",
"dob": "19880101",
"phones": ["555-123-4567", "555-555-5555"]
}
}
into an XML string that looks like this:
<user>
<name>John Doe</name>
<dob>19880101</dob>
<phones>555-123-4567</phones>
<phones>555-555-5555</phones>
</user>
A "Deserialize XML" action, when run on the above XML, would convert the XML into an object whose keys can be referenced in subsequent steps.
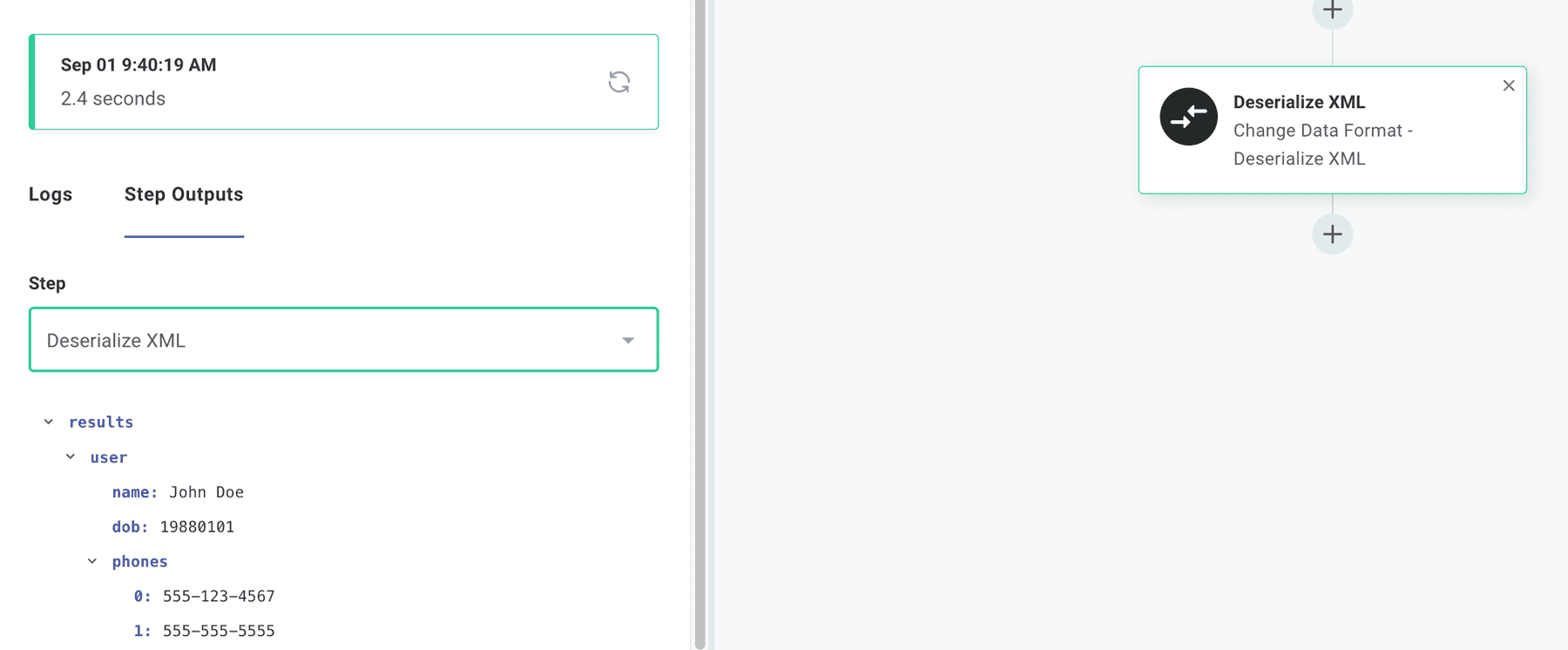
Actions
Convert To Boolean
Convert a value to a boolean | key: convertToBoolean
Input | Notes |
---|---|
The value to be converted to a boolean string / Required value |
Convert To Integer
Convert a value to an int | key: convertToInt
Input | Notes |
---|---|
The value to be converted to a integer string / Required value |
Convert To Number
Convert a value to a number | key: convertToNumber
Input | Notes |
---|---|
The value to be converted to a number string / Required value |
Convert To String
Convert a value to a string | key: convertToString
Input | Notes |
---|---|
The value to be converted to a string string / Required value |
CSV to JSON
Convert CSV to JSON | key: csvToJson
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | CSV data to convert to JSON | ||
CSV Header boolean isHeader | true | Specify if your CSV contains a header row. | |
Un-Flatten CSV Keys boolean / Required unFlattenCsvKeys | false | When enabled, keys with double-underscores will be parsed as nested objects. For example, 'person__first,person__last' will become '{ person: { first, last } }' rather than '{ person__first, person__last }' |
Example Payload for CSV to JSON
{
"data": "{\n \"person\": {\n \"first\": \"Bob\",\n \"last\": \"Johnson\"\n },\n \"dob\": \"1990-01-01\"\n}",
"contentType": "application/json"
}
CSV to XML
Convert CSV to XML | key: csvToXml
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | CSV data to convert to XML | ||
CSV Header boolean isHeader | true | Specify if your CSV contains a header row. | |
Un-Flatten CSV Keys boolean / Required unFlattenCsvKeys | false | When enabled, keys with double-underscores will be parsed as nested objects. For example, 'person__first,person__last' will become '{ person: { first, last } }' rather than '{ person__first, person__last }' |
Example Payload for CSV to XML
{
"data": "<dob>1990-01-01</dob>\n<person>\n <first>Bob</first>\n <last>Johnson</last>\n</person>",
"contentType": "text/xml"
}
CSV to YAML
Convert CSV to YAML | key: csvToYaml
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | CSV data to convert to YAML | ||
CSV Header boolean isHeader | true | Specify if your CSV contains a header row. | |
Un-Flatten CSV Keys boolean / Required unFlattenCsvKeys | false | When enabled, keys with double-underscores will be parsed as nested objects. For example, 'person__first,person__last' will become '{ person: { first, last } }' rather than '{ person__first, person__last }' |
Example Payload for CSV to YAML
{
"data": "---\nperson:\n first: Bob\n last: Johnson\ndob: '1990-01-01'",
"contentType": "text/yaml"
}
Deserialize BINARY
Deserialize BINARY data | key: deserializeFromBinary
Input | Notes |
---|---|
Data code / Required data | BINARY text to deserialize so it can be referenced in a subsequent step. |
Example Payload for Deserialize BINARY
{
"data": {
"person": {
"first": "Bob",
"last": "Johnson"
},
"dob": "1990-01-01"
}
}
Deserialize CSV
Deserialize CSV data | key: deserializeFromCsv
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | CSV text to deserialize so it can be referenced in a subsequent step. | ||
CSV Header boolean isHeader | true | Specify if your CSV contains a header row. | |
Un-Flatten CSV Keys boolean / Required unFlattenCsvKeys | false | When enabled, keys with double-underscores will be parsed as nested objects. For example, 'person__first,person__last' will become '{ person: { first, last } }' rather than '{ person__first, person__last }' |
Example Payload for Deserialize CSV
{
"data": {
"person": {
"first": "Bob",
"last": "Johnson"
},
"dob": "1990-01-01"
}
}
Deserialize JSON
Deserialize JSON data | key: deserializeFromJson
Input | Notes | Example |
---|---|---|
Data code / Required data | JSON text to deserialize so it can be referenced in a subsequent step. |
Example Payload for Deserialize JSON
{
"data": {
"person": {
"first": "Bob",
"last": "Johnson"
},
"dob": "1990-01-01"
}
}
Deserialize JSON Lines (.jsonl)
Transform .jsonl data to a JavaScript array | key: deserializeJsonl
Input | Notes | Example |
---|---|---|
JSONL Data code / Required jsonl |
Deserialize URL-encoded Form Data
Deserialize Form Data (x-www-form-urlencoded) | key: deserializeFormData
Input | Notes | Example |
---|---|---|
Data string / Required data | Form data to deserialize so it can be referenced in a subsequent step. | foo=bar&baz=123 |
Example Payload for Deserialize URL-encoded Form Data
{
"data": {
"foo": "bar",
"baz": "123"
}
}
Deserialize XML
Deserialize XML data | key: deserializeFromXml
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | XML text to deserialize so it can be referenced in a subsequent step. | ||
Parse numbers as strings? boolean numbersAsStrings | false | Interpret numbers as strings? |
Example Payload for Deserialize XML
{
"data": {
"person": {
"first": "Bob",
"last": "Johnson"
},
"dob": "1990-01-01"
}
}
Deserialize YAML
Deserialize YAML data | key: deserializeFromYaml
Input | Notes | Example |
---|---|---|
Data code / Required data | YAML text to deserialize so it can be referenced in a subsequent step. |
Example Payload for Deserialize YAML
{
"data": {
"person": {
"first": "Bob",
"last": "Johnson"
},
"dob": "1990-01-01"
}
}
JavaScript Object to CSV
Convert JavaScript Object to CSV | key: binaryToCsv
Input | Notes |
---|---|
Data code / Required data | JavaScript Object data to convert to CSV |
Example Payload for JavaScript Object to CSV
{
"data": "\"person__first\",\"person__last\",\"dob\"\n\"Bob\",\"Johnson\",\"1990-01-01\"",
"contentType": "text/csv"
}
JavaScript Object to JSON
Convert JavaScript Object to JSON | key: binaryToJson
Input | Notes |
---|---|
Data code / Required data | JavaScript Object data to convert to JSON |
Example Payload for JavaScript Object to JSON
{
"data": "{\n \"person\": {\n \"first\": \"Bob\",\n \"last\": \"Johnson\"\n },\n \"dob\": \"1990-01-01\"\n}",
"contentType": "application/json"
}
JavaScript Object to XML
Convert JavaScript Object to XML | key: binaryToXml
Input | Notes |
---|---|
Data code / Required data | JavaScript Object data to convert to XML |
Example Payload for JavaScript Object to XML
{
"data": "<dob>1990-01-01</dob>\n<person>\n <first>Bob</first>\n <last>Johnson</last>\n</person>",
"contentType": "text/xml"
}
JavaScript Object to YAML
Convert JavaScript Object to YAML | key: binaryToYaml
Input | Notes |
---|---|
Data code / Required data | JavaScript Object data to convert to YAML |
Example Payload for JavaScript Object to YAML
{
"data": "---\nperson:\n first: Bob\n last: Johnson\ndob: '1990-01-01'",
"contentType": "text/yaml"
}
JSON to CSV
Convert JSON to CSV | key: jsonToCsv
Input | Notes | Example |
---|---|---|
Data code / Required data | JSON data to convert to CSV |
Example Payload for JSON to CSV
{
"data": "\"person__first\",\"person__last\",\"dob\"\n\"Bob\",\"Johnson\",\"1990-01-01\"",
"contentType": "text/csv"
}
JSON to XML
Convert JSON to XML | key: jsonToXml
Input | Notes | Example |
---|---|---|
Data code / Required data | JSON data to convert to XML |
Example Payload for JSON to XML
{
"data": "<dob>1990-01-01</dob>\n<person>\n <first>Bob</first>\n <last>Johnson</last>\n</person>",
"contentType": "text/xml"
}
JSON to YAML
Convert JSON to YAML | key: jsonToYaml
Input | Notes | Example |
---|---|---|
Data code / Required data | JSON data to convert to YAML |
Example Payload for JSON to YAML
{
"data": "---\nperson:\n first: Bob\n last: Johnson\ndob: '1990-01-01'",
"contentType": "text/yaml"
}
Pretty Print
Format data to be more human-readable | key: prettyPrint
Input | Notes |
---|---|
Data text / Required data | Data to pretty print |
Serialize JSON Lines (.jsonl)
Serialize an array of JavaScript objects into .jsonl | key: serializeJsonl
Input | Notes |
---|---|
Array of JavaScript Objects to serialize string / Required array | Must be a reference to an array of JavaScript objects |
Serialize URL-encoded Form Data
Serialize Form Data (x-www-form-urlencoded) | key: serializeFormData
Input | Notes |
---|---|
Data code / Required data | Form data to deserialize so it can be referenced in a subsequent step. |
Example Payload for Serialize URL-encoded Form Data
{
"data": "foo=bar&baz=123"
}
XML to CSV
Convert XML to CSV | key: xmlToCsv
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | XML data to convert to CSV | ||
Parse numbers as strings? boolean numbersAsStrings | false | Interpret numbers as strings? |
Example Payload for XML to CSV
{
"data": "\"person__first\",\"person__last\",\"dob\"\n\"Bob\",\"Johnson\",\"1990-01-01\"",
"contentType": "text/csv"
}
XML to JSON
Convert XML to JSON | key: xmlToJson
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | XML data to convert to JSON | ||
Parse numbers as strings? boolean numbersAsStrings | false | Interpret numbers as strings? |
Example Payload for XML to JSON
{
"data": "{\n \"person\": {\n \"first\": \"Bob\",\n \"last\": \"Johnson\"\n },\n \"dob\": \"1990-01-01\"\n}",
"contentType": "application/json"
}
XML to YAML
Convert XML to YAML | key: xmlToYaml
Input | Default | Notes | Example |
---|---|---|---|
Data code / Required data | XML data to convert to YAML | ||
Parse numbers as strings? boolean numbersAsStrings | false | Interpret numbers as strings? |
Example Payload for XML to YAML
{
"data": "---\nperson:\n first: Bob\n last: Johnson\ndob: '1990-01-01'",
"contentType": "text/yaml"
}
YAML to CSV
Convert YAML to CSV | key: yamlToCsv
Input | Notes | Example |
---|---|---|
Data code / Required data | YAML data to convert to CSV |
Example Payload for YAML to CSV
{
"data": "\"person__first\",\"person__last\",\"dob\"\n\"Bob\",\"Johnson\",\"1990-01-01\"",
"contentType": "text/csv"
}
YAML to JSON
Convert YAML to JSON | key: yamlToJson
Input | Notes | Example |
---|---|---|
Data code / Required data | YAML data to convert to JSON |
Example Payload for YAML to JSON
{
"data": "{\n \"person\": {\n \"first\": \"Bob\",\n \"last\": \"Johnson\"\n },\n \"dob\": \"1990-01-01\"\n}",
"contentType": "application/json"
}
YAML to XML
Convert YAML to XML | key: yamlToXml
Input | Notes | Example |
---|---|---|
Data code / Required data | YAML data to convert to XML |
Example Payload for YAML to XML
{
"data": "<dob>1990-01-01</dob>\n<person>\n <first>Bob</first>\n <last>Johnson</last>\n</person>",
"contentType": "text/xml"
}