Fetching Step Results Programmatically
When you invoke an integration asynchronously, an executionId
is returned:
curl https://hooks.prismatic.io/trigger/EXAMPLE== \
--data '{}' \
--header "Content-Type: application/json"
{"executionId":"SW5zdGFuY2VFeGVjdXRpb25SZXN1bHQ6OTdiNWQxYmEtZGUyZi00ZDY4LWIyMTgtMDFlZGMwMTQxNTM5"}
You can use the executionResult along with that executionId
to fetch the results of that execution.
query ($executionId: ID!) {
executionResult(id: $executionId) {
startedAt
endedAt
error
stepResults (stepName: "codeBlock") {
nodes {
stepName
resultsUrl
}
}
}
}
{
"executionId": "SW5zdGFuY2VFeGVjdXRpb25SZXN1bHQ6OTdiNWQxYmEtZGUyZi00ZDY4LWIyMTgtMDFlZGMwMTQxNTM5"
}
If the execution is still running, the endedAt
property will be null
.
You can query for stepResults
, and specifically ask for the resultsUrl
of a specific step.
Step names are "camelCased" in the stepResults
filter (so, My Code Block
-> myCodeBlock
).
Step results are written out to Amazon S3, and the resultsUrl
returns a short-lived presigned URL where you can fetch the step's results.
A step can return anything.
They often return JavaScript objects or JSON, but some steps return binary files such as PDFs or MP3s or a JavaScript Buffer
.
To account for the variety of things that a step can return, Prismatic uses msgpack to package up step results.
The step result file you download from S3 will appear encoded, but after "unpacking" the contents, it'll look like normal JSON (or the type of data your step yielded).
To "unpack" step results, use msgpack.decode()
in Node.js (or a similar function in a language of your choosing - msgpack
supports many languages).
Fetching and unpacking step results with Node.js
In the example below, assume that a step named Create Object
returned a JavaScript object with three properties: myImage1
, myImage2
, and myJavaScriptObject
.
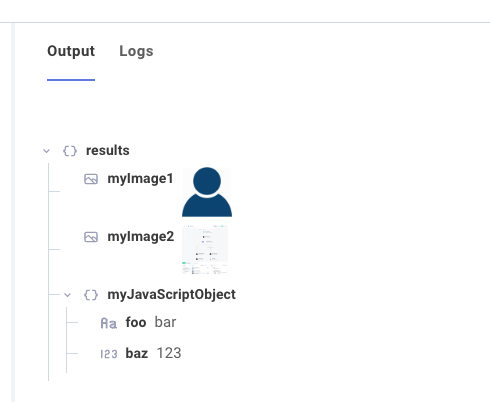
The first two properties are images, and the third is a JavaScript object. This code will fetch the step results, decode them, and write the images and JavaScript object to disk. The JavaScript object will be encoded as JSON.
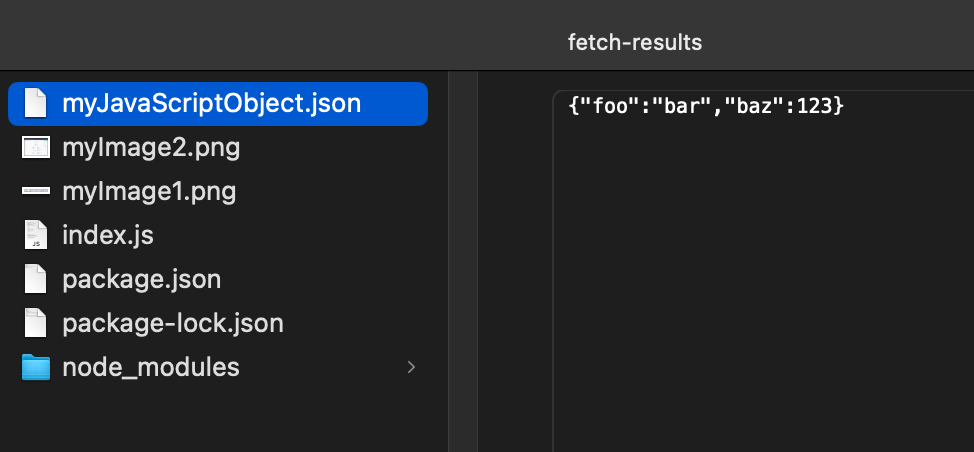
const msgpack = require("@msgpack/msgpack");
const fs = require("fs");
const PRISMATIC_URL =
process.env.PRISMATIC_URL || "https://app.prismatic.io/api";
const PRISMATIC_API_KEY = process.env.PRISMATIC_API_KEY;
const query = `query getStepResults ($executionId: ID!) {
executionResult(id: $executionId) {
startedAt
endedAt
error
stepResults(displayStepName: "Create Object") {
nodes {
stepName
resultsUrl
}
}
}
}`;
async function main() {
const executionId = process.argv[2];
// Make an API call to Prismatic to get the results URL
const apiResponse = await fetch(PRISMATIC_URL, {
method: "POST",
headers: {
"Content-Type": "application/json",
Authorization: `Bearer ${PRISMATIC_API_KEY}`,
},
body: JSON.stringify({
query,
variables: {
executionId,
},
}),
});
const apiResult = await apiResponse.json();
const resultUrl =
apiResult.data.executionResult.stepResults.nodes[0].resultsUrl;
// Get the result from Amazon S3
const encodedResult = await fetch(resultUrl);
const encodedResultBuffer = await encodedResult.arrayBuffer();
// Use msgpack to decode the results
const decodedResult = msgpack.decode(encodedResultBuffer);
const { myImage1, myImage2, myJavaScriptObject } = decodedResult.data;
// Write the results to disk
fs.writeFileSync("myImage1.png", myImage1.data);
fs.writeFileSync("myImage2.png", myImage2.data);
fs.writeFileSync(
"myJavaScriptObject.json",
JSON.stringify(myJavaScriptObject),
);
}
main();